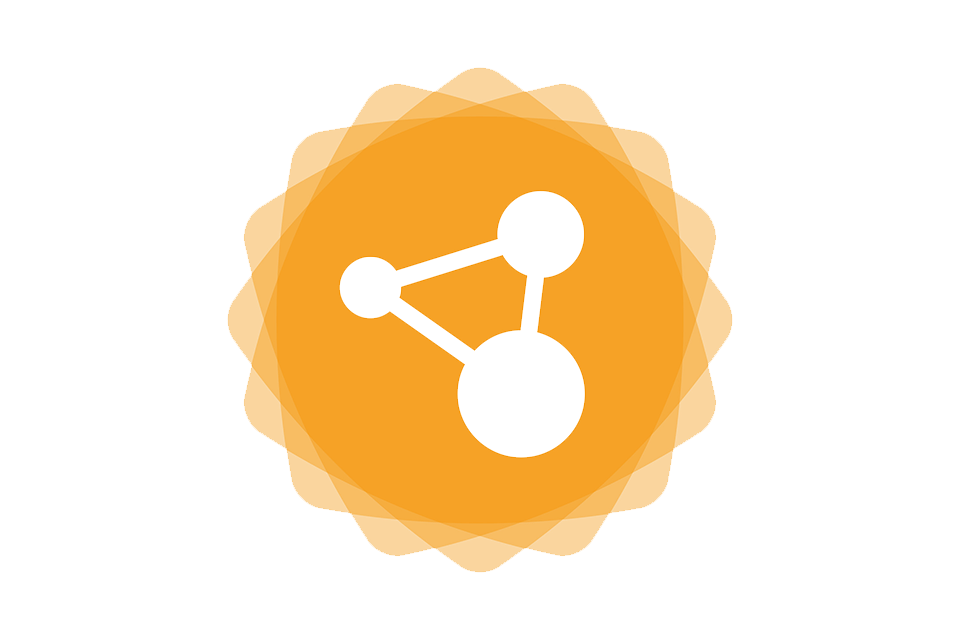
Native sharing centers are available both on iOS and Android. If you are wondering what is a native sharing center, it is what appears when you click on sharing icons in apps.
Usually, clicking on a sharing icon opens a standard panel with standard sharing actions: email, Facebook, open in a browser…
Apps can add their own sharing methods to these panels. For example, if you have installed Slack or Whatsapp, you will be able to share links and photos with these apps using the native share capabilities. The native sharing user experience is very smooth – compared to the usual web sharing – as the user is already connected with his/her account.
In this tutorial, we are going to learn how to leverage the native sharing centers in our apps.
Add Social Sharing Cordova Plugin
First we have to add the capability to call the native sharing center using a Cordova plugin. We are going to use the Social Sharing plugin done by Eddy Verbruggen.
To add this plugin to your app:
- Edit your app by clicking it in the applications list
- Go to the PhoneGap Build box
- Go to the PhoneGap configuration
- Paste the following tag in the Plugins text area:
<plugin name=”cordova-plugin-x-socialsharing” source=”npm” />.
Depending on which PhoneGap Build cli version you are using, you may have to force the socialsharing plugin version: if you have an error when building the app, try to force version like so:
<plugin name=”cordova-plugin-x-socialsharing” source=”npm” spec=”5.4.1″ />
From now the sharing plugin will be included in your app when compiling and you will have access to the JavaScript API it provides.
Add Sharing Button To Your App
We are going to add a simple share button to the single template of the default Q theme. It will call the native sharing center with pre-defined parameters.
Note: instead of a link, we recommend that you use the default iOS and Android icons as users already know them. You will easily find SVG version of each (eg. here’s one for Android and this one for iOS).
Add Share Button To Single Template
- In your theme’s folder, open single.html
- Add the following tag in the single-content div
<div id="single-content" class="single-content"> <button id="share-button" class="share-button">Share</button> <%= post.content %> </div
Store Post Title And URL
To share a post from the app, we use the shareWithOptions() function provided by the Social Sharing plugin. This function expects parameters such as a message, an URL back to the post… In this example, we are going to share the post title and its original URL (ie. the URL to the article on the website). These parameters will be stored as custom attributes of the share button when a post is displayed.
- Open functions.js in your theme’s folder
- Go to the screen:showed event
- First, we have to grab the current screen object
App.on( 'screen:showed',function( current_screen,view ){ var currentScreenObject = App.getCurrentScreenObject();
- Now we can get the permalink and title values to set our custom attributes when a post is displayed (still in screen:showed event) :
if (current_screen.screen_type == "single" || current_screen.screen_type=="page") { $( '#share-button' ).attr( 'data-url', currentScreenObject.permalink ); $( '#share-button' ).attr( 'data-title', currentScreenObject.title ); //Comment the following if you don't need to share post featured image: $( '#share-button' ).attr( 'data-thumbnail', currentScreenObject.thumbnail && currentScreenObject.thumbnail.src ? currentScreenObject.thumbnail.src : '' );
- As we don’t want to keep these values, we can clean them when leaving the screen, adding the following line to the screen:leave event (only useful if you place the share button outside post content: in top navigation bar for example)
if ( current_screen.screen_type == 'single' ) { // Unset data necessary for sharing $( '#share-button' ).attr( 'data-url', '' ).attr( 'data-title', '' ).attr( 'data-thumbnail', '' );
Add Sharing Function
Add the following function at the end of your functions.js code (but still inside the main file’s function(), so before the final “});” )
// Finger releases the share button function shareButtonTapOff( e ) { e.preventDefault(); // Get data to be shared var shareUrl = null; if ( $( this ).attr( 'data-url' ) != '' ) { shareUrl = $( this ).attr( 'data-url' ); } var shareMessage = "I've just discovered a great article and I think it may interest you"; if ( shareUrl != null ) { shareMessage += ": " + shareUrl; } else { shareMessage += '.'; } var shareSubject = null; if ( $( this ).attr( 'data-title' ) != '' ) { shareSubject = $( this ).attr( 'data-title' ); } var shareThumbnail = null; //Comment the following if you don't need to share post featured image: if ( $( this ).attr( 'data-thumbnail' ) != '' ) { shareThumbnail = $( this ).attr( 'data-thumbnail' ); } // Launch OS sharing center (and check if the necessary Phonegap plugin is available - https://github.com/EddyVerbruggen/SocialSharing-PhoneGap-Plugin/) try { window.plugins.socialsharing.share( shareMessage, // Message shareSubject, // Subject shareThumbnail, // Image shareUrl, // Link function( result ) { /*alert( 'Success' );*/ }, // Success feedback function( result ) { /*alert( 'Failed' );*/ } // Error feedback ); } catch( err ) { console.log( "Sharing plugin is not available - you're probably in the browser" ); } }
Trigger The Sharing
Everything is in place, now we need to bind our sharing function to the Share button by adding the following code to functions.js just after the function added in the previous section.
// Share button events $( "#app-layout" ).on( "touchend", "#share-button", shareButtonTapOff );
And we’re done! Now users can share an article using the native OS sharing center.
Happy coding 🙂
Hello guys am a fan, hope some day can have half your skills.
Am having problem add social share to the app i don’t know what’s is the problem but i guess it’s the php i create a folder named php and drop the php file with the code in it, i was confused cuz in the tutorial seem like it should have a php dir by default but i couldn’t find it plz help me 🙂
Hi Bruno,
The code described in this tutorial is Javascript, not PHP. It goes into the functions.js file of your WP-AppKit app theme (/wp-content/themes-wp-appkit/q-android for example).
If you need to add some PHP code from another tutorial, it will go in the app theme “php” folder (you can see there is one “php” folder in /wp-content/themes-wp-appkit/q-android).
For any further questions, please contact us at support@uncategorized-creations.com.
Regards,
Hi there , just i want to notice that there is Syntax error in the add sharing function snippet between lines 38 and 39 , thanks .
Thanks 🙂 Fixed.
Hi, thanks for this great article. It runs smoothly. I have a huge doubt. My application is private and I would like to share the title and the content, the link I do not need it.
Thanks for the help and for this great tool.
Hi and thanks for the feedback,
If you don’t need the link, have you tried to pass an empty “data-url”:
$( '#share-button' ).attr( 'data-url', '' );
?
Does this work for you?
Hello,
The implemented button works and executes the sharing function, but at the moment of sending it I only look at a “.” and I do not see the title.
can you help me with this please?
Thank you
Hi Alfredo, can you please write to support[at]uncategorized-creations.com joining the parts of your app theme (Javascript and template) where you implement the sharing feature, so that we can try to narrow down what’s causing your issue?
Thanks
Hello, I would like to use this function only to download the download link of my application and possibly a message with, but I can not do that, can you help us?
Hi, you can pass your download link and message in the shareMessage variable used in the code of this tutorial.
If you need more help, please write us to support[at]uncategorized-creations.com describing what you need exactly so that we can try to help you.
Regards
Hello, thank you, I managed to do what I wanted by following your indication
Hello:
Congratulations on your plugin
The implemented button works and executes the sharing function. But when it is sent, the title, url and the image do not appear.
Thank you
Hi Manuel,
Hard to say what can be wrong in your case. Maybe the socialsharing plugin is not correctly added to your app?
Could you please write to support[at]uncategorized-creations.com with some more information about your Phonegap version, Cordova plugins versions and what happens exactly when you click the share button inside your compiled app? Thanks.
Regards