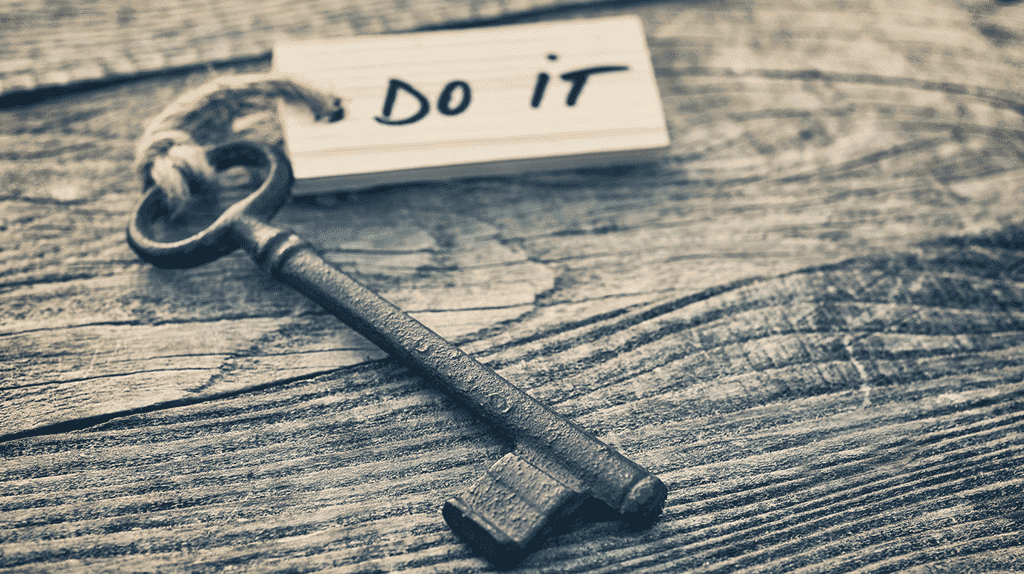
As of version 0.4, WP-AppKit handles secured user authentication that allows to:
- log in WordPress users from you app,
- restrict some part of your app content to authenticated users with given permissions,
- post comments from your app,
- make authenticated queries to WordPress,
- secure download links,
- and any other cool stuff you can think of when you can authenticate users from apps.
WP-AppKit leverages the RSA public key encryption to encrypt all sensible data (password at handshake for example) and secret key based HMAC controls for all authenticated communications between the app and the WordPress server.
Here are the basic steps that will bring user authentication to your WP-AppKit app:
Create a RSA Private Key
On Windows the easiest way is to use PuTTY (see the private key generation doc for detailed steps).
On iOS/Unix based systems, simply use the “openssl” command:
openssl genrsa -out rsa_1024_priv.pem 1024
Then, open the generated private key file (rsa_1024_priv.pem) in a text editor, and copy its content to your clipboard. This is your private key, which should be something like:
-----BEGIN RSA PRIVATE KEY----- MIICWgIBAAKBgQCoLQvcggQflUc5ug9Ti10tcrKr9DVn/huAZquQ+XrjLBdJMXC/ l3mcL+J7svjbr0+f+JAPrUeSFB3yiGiIISLDxsbQmDaujKkZT+yTXfJ3uJFjppNP Bdbk626MbPnRUKoWgMPlAxjYemoBV6Tg9AFcC2q8oCUD+SDbpKR2rfddHwIBJQKB gCRcxEtaYcGjvF+CLNPLGxC+2oYLSdFLsutUeB9YiUXgBQjoGGDGzi+bppdJSpBd QaYMOtL1CI5Jie9AFpn5U5+ZRSobz4v3RgCHBkvZsbAP1ararV1yCuDFyYIbQRg/ +pYltCfctB0teRiIq6j8fz07/gh/bElTdPA5mT2dJLGtAkEA6ZkqhIN4MOp9SGSZ pqJPrR8qZ3NBNM04LlHg4rrnk7kZJfjWeS2iuKX4e8RfsAEhV5ZuRnpGxRs7vntg ba5+PwJBALhNw1MTTwQa2ZcK2uh6WcI2Awu+PaGXXSpYscwx2ZUKBuxp1i0qLs9z 92PGk7Qzv35dZwxV6hIIyNvEWUl/KSECQEvC+Qhh/Xel/x5l0PfF8FPSwaUCyQo0 uEZfvo65KILv7HsEmJYA9oETO9UdGB1oJn1Fi4x6rysrbVKI4QD6UnUCQHeMYwV0 Tu3n5xXdhxNWSA9FoRxfuUY4qyJjC4temvjj6NCzWn4pMx7HwxBJdJCQRNxYQteR rJYTiTSbCXvIGq0CQQDVnF8XYGUGjL16R0VbSOjmpizAtEQNDguLTPpHPP6w8UC3 m8NgYZ+Ht3+D1YsQ7zbc9M0J8XrfU1tiXnoQZZrl -----END RSA PRIVATE KEY-----
Link the Private Key to Your App
In your WordPress Back Office, go edit your App and paste your private key into the “Authentication Settings” > “App Private Key” field:
Save your app. It is now ready for secured user authentication.
Log Your Users In
To authenticate users from your app’s theme, you’ll use the WP-AppKit User Authentication JS API (JS module used as Auth var in the following examples).
Here is a login example (in the theme’s functions.js), where we suppose that the theme includes a login form (#login-form) where the user fills in his/her login (input#userlogin) and password (input#userpass), and submits with a #go-login button:
//In functions.js define( [ ..., 'core/modules/authentication', ... ], function( ..., Auth, ... ) { $( '#login-form' ).on( 'click', '#go-login', function( e ) { e.preventDefault(); //Log the user in! Auth.logUserIn( $('#userlogin').val(), //from form text input#userlogin $('#userpass').val(), //from form password input#userpass function( user_data_and_permissions ) { //Do something when authentication went ok }, function( error) { //Do something when authentication failed //(wrong login, wrong password etc) } ); } ); } );
Then you can retrieve the currently logged in user:
var current_user = Auth.getCurrentUser();
check the WordPress user’s capabilities:
if ( Auth.currentUserCan( 'premium_access' ) ) { ... }
log the user out:
Auth.logUserOut();
And many other things that you’ll find in the doc 🙂
To display user data in your templates, add the user object to your template vars using the “template-args” filter :
//In functions.js App.filter( 'template-args', function( template_args, view_type, template ) { if( template == 'my-user-page' ) { template_args.user = Auth.getCurrentUser(); } return template_args; } );
Then, the currently logged in user object is available as “user” var in your “my-user-page.html” template:
//In "my-user-page.html" template: User login: <% user.login %><br> User capabilities: <ul> <% _.each( user.permissions.capabilities, function( capability ){ %> <li><%= capability %></li> <% } ); %> </ul>
You can also check a complete implementation example in the “User Authentication Demo” version of our Q for Android theme.
Happy user authentication folks!
This looks good and I have been following WP app kit.
I’m curious to know why can’t I do the same with the soon to be native Rest API?
You can and… we will 🙂 But not until the REST API is fully integrated to the core and stable. Apps are not updated as easily as web sites and any change to the API they rely on means updates on the app’s side. Note that WP-AppKit is more than an API, it’s also a JS framework to build app themes, enhancement to the admin, project export for PhoneGap Build, etc.
Is it possible to add push notification function using WP APPKit?
Thank you Tylor.
We’re currently working on (native) push notifications. First thing we’ve done is to add deeplinks to WP-AppKit. The idea is to able to have a custom URL scheme to open any screen in the app. That’s the first step for push notifications as you usually need to deeplink to your app from push. Deeplinks will be available with WP-AppKit 0.5.
Second step is to be able to let users register for push notifications and actually to be able to send push notifications. For that, we’ll use the PushWoosh service as we don’t intend to create that kind of service. PushWoosh has a nice Cordova integration and we’ll build an addon to support it in WP-AppKit (yes you can create addons for WP-AppKit). We’re going to release this addon with the 0.6 probably along with an addon marketplace.
It’s amazing! Thanx for great work! Maybe in next release WP-AppKit you planning add user regiter support?
Yes. That’s something on our roadmap. We may release that for 0.6.
Great! And second question, maybe you planing use in template some famous framework like Foundation frontend, onsenui or ionic? Bottstrap template looks good, but not better for mobile app. Don’t have sliding menu like Foundation.
Well the magic lies in the fact you may use whatever you like as a JS framework for your themes. You may have notice that our default themes don’t even use a framework 😉 iOnic is another beast as it requires AngularJS. You won’t be able to use it with WP-AppKit.
I try framework with AngularJS – fail (((. Try Foundation
Hello thank you very much. It worked incredible. I have a problem, I need to login to several devices. When you log in to another device, the session on the first device is closed. Thanks for the help. Excellent work.
Hi Victor and thanks for the nice feedback.
Yes, this is the default behavior for now, with current version of user authentication, a user can only login on one device at a time.
I’ll take a look at it in the coming days to see if we can find a way to allow multi logins, and I’ll come back to you.
Regards
Hello, thank you very much for the response. We will have news about the login on different devices?
On Thursday I have an event and I would like to leave this detail ready. If necessary, we can contribute economically to this situation.
We are customers and we have bought the addons.
Thank you very much, Regards.
Hi Victor,
I have started to implement this multiple login feature.
You can follow development in this issue on github: https://github.com/uncatcrea/wp-appkit/issues/378.
I’ve pushed a first implementation on our development branch and it is already working and can be tested, either by retrieving (clone or download) the development branch of WP-AppKit, or simply by replacing in your local install the only file that changed for now: https://github.com/uncatcrea/wp-appkit/blob/development/wp-appkit/lib/user-permissions/auth-engines/rsa-public-private-auth.php.
You can test it now and give us some feedback 🙂
For convenience, please post your feedbacks or next questions directly in the github issue 🙂
Regards