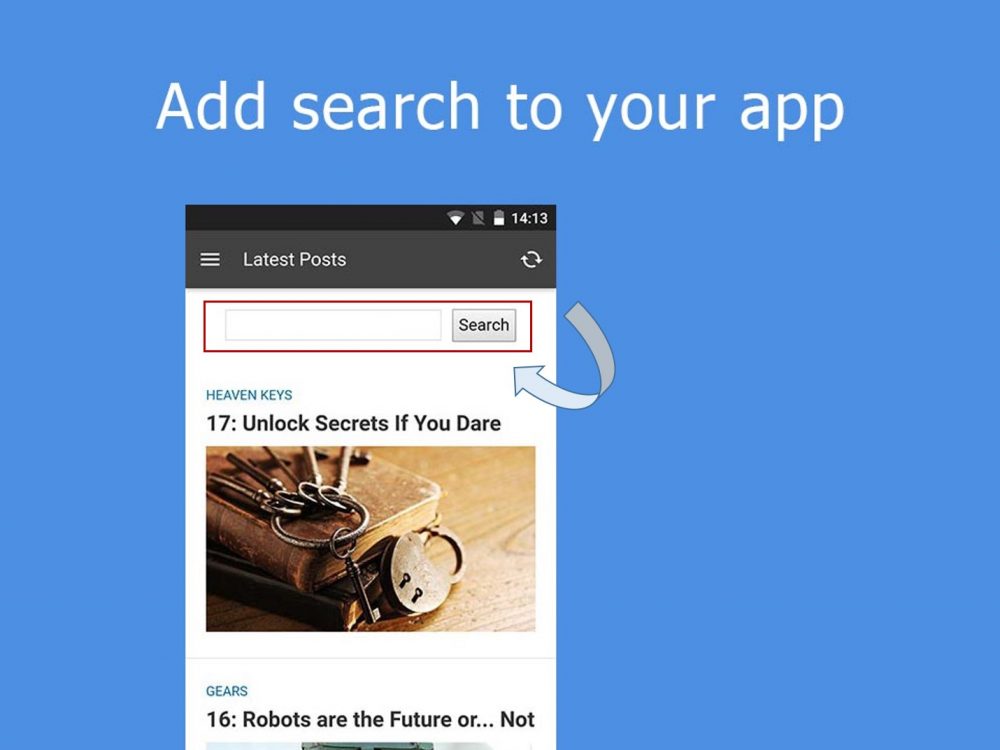
With WordPress, we’re used to have a search box in our themes. But how do we get this feature for our apps? In this tutorial, we’re going to see how to add a search box to our WP-AppKit apps. This search will leverage the WordPress native search. We’ll be able to type keywords in a search field, send the request to the server where WordPress lives and get results in return. It is a basic search but it will help you to understand how to implement that kind of feature in your apps.
Good to know: I am going to use the pre-installed Q for Android theme. (Of course you can use your own theme.) So you may want to duplicate Q for Android in order to modify it safely.
Add a Search Field To The Theme
Our search field will be located at the top of the result lists. So we have to modify the archive template.
Open the archive.html file located at the root of your theme’s folder with your favorite editor, and add the following HTML code:
<div class="search-container"> <form id="search-form"> <input value="<%= current_search.search_string %>" id="search-field" class="search-field" type="search" /><button id="search-button" class="search-button">Search</button> </form> </div>
This code simply adds a search input and a search button.
Let’s add also a bit of styling, nothing fancy just the minimum. Open the post-list.css file in the css subfolder of your theme and add the following rules:
/* * Search */ .search-container { padding: 20px 5px 20px 5px; text-align: center; } .search-field { width: 60%; height: 30px; line-height: 20px; font-size: 16px; margin-right: 10px; padding: 2px; } .search-button { padding: 4px; line-height: 20px; font-size: 16px; }
Again nothing fancy, just the minimum to get a decent search field.
Good to know: using a search input instead of a text input allows to trigger the search dedicated keyboard on mobile. It also provides a small cross in the field to empty the search field.
Implement Search
Now, let’s get serious. we’re going to develop the search itself (ie. sending a search request to the server and get results in return).
On the App Side
Open the functions.js file in the js subfolder of your theme. You can place the sample code at the end of the file.
First we need to store current search terms using a basic object (it could be a variable):
/** * Memorize current search so that it is always available, * even after changing screen */ var current_search = { search_string: '' };
Then we can send our search request when clicking the search button.
So we bind the function on the button’s click event (and prevent the default behavior),
$( '#app-layout' ).on( 'click', '#search-button', function( e ) { e.preventDefault(); } );
Get the search terms we may have in the search field,
$( '#app-layout' ).on( 'click', '#search-button', function( e ) { e.preventDefault(); //Set search params from HTML form: current_search.search_string = $('#search-field').val().trim(); } );
And refresh the list to display the search result.
$( '#app-layout' ).on( 'click', '#search-button', function( e ) { e.preventDefault(); //Set search params from HTML form: current_search.search_string = $('#search-field').val().trim(); //Get updated data from server for the current component: App.refreshComponent({ success: function( answer, update_results ) { //Server answered with a filtered list of posts. //Reload current screen to see the result: App.reloadCurrentScreen(); }, error: function( error ) { //Maybe do something if filtering went wrong. //Note that "No network" error events are triggered automatically by core } }); } );
We also have to send any search terms we may have to the web service. For that we use the web-service-params filter.
/** * Add our search params to web services that retrieve our post list. * Applies to "Live Query" web service (that retrieves filtered component's post list) * and to "Get More Posts" web service (so that search filters apply to pagination too). */ App.filter( 'web-service-params', function( web_service_params ) { //If the user provided non empty search params: if( current_search.search_string !== '' ) { //Add search params to the data sent to web service: web_service_params.my_search_filters = current_search; //Those params will be retrieved with WpakWebServiceContext::getClientAppParam( 'my_search_filters' ) //on server side. } return web_service_params; } );
Last thing we’ll do on the app’s side is to ensure that search terms are still displaying in the search field after the results come back from the server. For that, we make current_search object available to the archive.html template using the template-args filter.
/** * Add * - current search params to the archive template, so that they're available in archive.html. */ App.filter( 'template-args', function( template_args, view_type, view_template ) { if ( view_type === 'archive' ) { template_args.current_search = current_search; } return template_args; } );
Then we can use template tags to catch the search terms on the template itself.
<div class="search-container"> <input value="<%= current_search.search_string %>" id="search-field" class="search-field" type="search" /><button id="search-button" class="search-button">Search</button> </div>
On the Server Side
At this stage of the tutorial, nothing will happen as we didn’t tell the server how to handle the search requests. We are going to do that by
- adding a .php file in the php subfolder of your theme (eg. search.php).
- using the wpak_posts_list_query_args hook to trigger the WordPress search when search terms are sent to the web service.
<?php /** * Basic search & filter on post lists. * Shows how to implement a search by string * See functions.js in your theme for the client side */ /** * Add search params sent by the app to the default component's query. */ add_filter( 'wpak_posts_list_query_args', 'search_component_query', 10, 2 ); function search_component_query( $query_args, $component ) { $my_search_filters = WpakWebServiceContext::getClientAppParam( 'my_search_filters' ); if ( !empty( $my_search_filters ) ) { if ( !empty( $my_search_filters[ 'search_string' ] ) ) { $query_args[ 's' ] = $my_search_filters[ 'search_string' ]; } //Note : default WP ordering for searchs is : // ORDER BY wp_posts.post_title LIKE '%search_string%' DESC, wp_posts.post_date DESC //which is not compatible with the default "Get more posts" feature that requires ordering by date. //Note: As of WP-AppKit 0.6, if you want to keep WP Search ordering, you can use the //'use-standard-pagination' filter on app side (which will switch back to standard WP pagination), //and comment the following line. $query_args[ 'orderby' ] = 'date'; } return $query_args; }
Now our search is complete 🙂 Let’s see how it works.
Again, this tutorial shows you how to implement a very basic search feature in your app. There’s many ways to enhance it like checking for no network situations or adding filters.
As usual if you have remarks or questions, you can use the comments below.
This is so awesome! First I want to thank you so much for WP-AppKit. I have tried many solutions for easily creating a mobile app version for my WP site and ever since I found this site I immediately switched over to WP-AppKit and I really really love it!.
After setting up WP-Appkit on my site and testing it on my phone I was just thinking how nice it would be to have a search box so people can easily find what they are looking for in the app. The only problem was I didn’t have enough coding knowledge to do so. But then guess what? Immediately a notification from chrome te pops up sayying “How To Add a Search Box To Your App – uncategorized-creations.com” now you have solved my problem. What a miracle! 🙂
So far I only understand HTML and CSS so I really want to learn Javascript and I have found this site to be really beneficial for me. Once again thank you so much for WP-AppKit and all the tutorials like this. It is so very very kind of you. Can’t wait for the next WP-AppKit awesomeness. Wish you all the best!
Thank you for your feedback. Hope that your JavaScript journey will be great 🙂
Thanks, maybe someday I will be able to create something that others can benefit from also just like you have done with WP-AppKit.
Btw, I just wanted you to know that I got it working on my app with some custom styling too.. Here’s what is looks like http://goo.gl/DwsSnV 🙂
Hi Benjamin,
Very nice functionality ! I get an error on line “error: function( error ) {” in function.js.
“Uncaught SyntaxError: Unexpected identifier”
I put js code at the end just before closing brakets of “define”. Can’t where is the error…
Jérôme
Hi, indeed a coma was missing just before error callback line 13 in the App.refreshComponent() example. It’s fixed now!
Thanks for your feedback 🙂
It works now like a charm. Nice work 🙂
This is really cool!
I was looking at a post on Github about integrating a search box.
And then here it is!
Will it only search the “frontpage” or can it search within posts or pages too?
What a great plugin so far!
Thank you 🙂 For the tutorial, the search box will be displayed on all lists (ie. archive.html template). Of course you may display it wherever you need (eg. menu, single…). The search itself is using the WordPress search.
Thanks for the reply Benjamin, so i could add the search function to “single”, and then be able to search a specific post with a lot of text?
If that is possible, im really excited about this!
When i add:
<input value="” id=”search-field” class=”search-field” type=”search” />Search
To Single.html – i get a lot of errors, and the single posts wont open.
I figured it out, i had to add the template arg hook.
The search bar is now present on single posts. However, i wont search the text.
If I understand correctly, you’d like to implement a kind of Ctrl+F search as we may have on desktop browsers, aren’t you? If so, it is a quite different type of search. The search I demonstrated in the tutorial searches the WordPress database. To build a Ctrl+F search, you’ll have to use JavaScript and parse the div where post text is (probably using a regexp).
Hi Benjamin,
Thats correct, our app only has 5 posts. So searching for a post title is kinda pointless when you can see them all on the screen anyway. But each of our posts has A LOT of content. So a search feature inside posts would be awesome. Kinda like CTRL+F as you mentioned.
But im not sure if thats possible, or at least i dont know how to make that!
It would require to develop a JavaScript function to search the post div content (probably with a bit of regexp too). We can’t work that out at the moment, having a lot of stuff on going but it is an interesting idea.
Thanks for this TUT really help.
Can you please do a TUT on how add sharing function to the App
Yes. I add that to the tut list ideas.
“Then we can use template tags to catch the search terms on the template itself.”
<div class=”search-container”>
<input value=”<%= current_search.search_string %>” id=”search-field” class=”search-field” type=”search” /><button id=”search-button” class=”search-button”>Search</button>
</div>
Can you tell me exactly where to add these lines of code ? There are already added to archive.html… What file are you calling the template ?
Excuse-me if it is a stupid question but I am not programmer at all…
I cut & paste each code block in mentioned file but I cannot have the search box appearing… I get or a blank page (if code block above is added to functions.js) or correct display but no search (if don’t add the code block above anywhere but archive.html).
Sorry again for newbies question and thanks for any help
Eric Collart
The line you’re refering to is an explanation of what the code inserted in archive.html does (catching the search string if there’s one). At this stage of the tutorial, nothing appears and it is normal. You have now to add the server side code to make it work.
Nice tutorial. Did anyone try to implement offline search?
Hi all,
I have followed the tuto step by step and rebuilded the App with Phonegap build but it doesn’t work at all.
Before rebuilding the search imput didn’t appear.
After rebuilding the App freezes and no post appears.
Any idea ? or working modifyed files with Android themes ?
Regards.
François.
Hi François,
Can you please contact us at support[at]uncategorized-creations.com so that we can try to find what’s causing your issue?
Regards