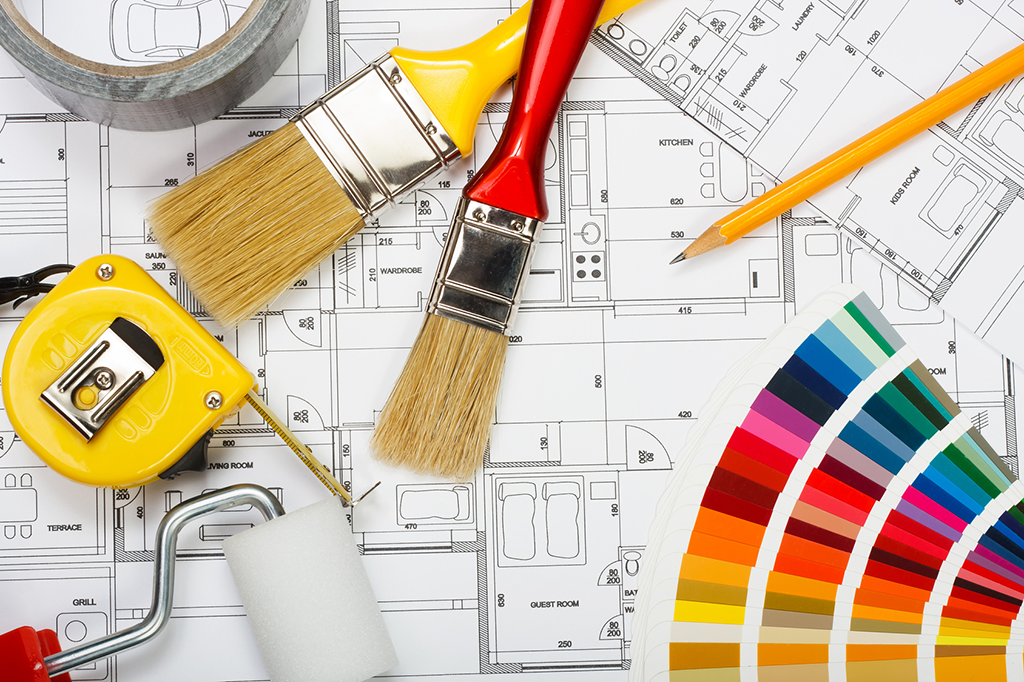
By default, each WP-AppKit app’s screen has a corresponding template associated to it:
- post lists render using the archive.html template
- post details render using the single.html template
- etc.
And each of those templates receives a set of pre-defined variables that you can use to display your screen data:
- in archive.html you can use list_title (the title of the post list screen), posts (array of posts to display), and total (total number of posts in the post list).
- in single.html you can use the post object that contains post’s title, content, date, featured image, etc.
But what if you want to go further than this default behavior?
Let’s see how you can define your own templates and customize the data that is passed to them.
Define a Specific Template For a Given Category
Using the archive.html template for all categories is so boring… Here’s how you can define you’re own custom template to render your “Special” category (slug “special-category”):
//In theme's functions.js for example: //Set our custom template using the 'template' filter: App.filter( 'template', function( template, current_screen ) { //Use the [your-theme]/special-category-template.html template //when rendering 'special-category' screen : if( TemplateTags.isCategory('special-category', current_screen) ) { template = 'special-category-template'; //Don't need .html here. } return template; } );
Then in your special-category-template.html you can use list_title, posts and total variables just as you would do in the default archive.html template.
In this example we’ve seen how to use the “template” filter to set a custom template for a category screen, but the same applies if you want to set a specific template for:
- a particular post screen: you would test if current_screen.item_id == my_post_id,
- a particular post type screen: test if TemplateTags.isPostType( ‘my-post-type’ )
- a particular component screen: test if current_screen.component_id == ‘my-component-slug’,
- and any other app screen you may think of…
See the doc to learn more about what you can do with the “template” filter.
And How Can I Pass Special Data To My Special Template ?
Of course your special category is linked to some special data (other than list_title, posts and total) that you would like to see available in your custom template.
That’s fair. Let’s see how you can handle this using the “template-args” filter:
The “template-args” filter is a pretty useful tool that allows to pass any data (numbers, strings, JSON Objects, JS modules) to any of your templates.
This applies to native templates (‘archive.html’, ‘single.html’, ‘layout.html’, ‘menu.html’ etc) and to any custom template you define.
Here we suppose that you had special_data (JSON object) stored in Local Storage (using the Persistent Storage module) and that you want to pass this data to your special-category-template.html template:
//In your theme's functions.js define( [ 'core/theme-app', 'core/modules/persistent-storage' ], function( App, PersistentStorage ) { App.filter( 'template-args', function( template_args, view_type, view_template ) { //Make "special_data" available in the "special-category-template.html" template: if( view_template == 'special-category-template' ) { template_args.special_data = PersistentStorage.get( 'special_data' ); } return template_args; } ); } );
Then in your special-category-template.html template you can now use “special_data“:
here we suppose that your special_data object contains special_data.first_thing (string) and special_data.second_thing (list of items):
//In [your-theme]/special-category-template.html <h1><%= list_title %></h1> <div class="my-special-data"> <p>First things first: <%= special_data.first_thing %></p> <div> And let the second thing be a list: <ul> <% _.each( special_data.second_thing, function( item ) { %> <li><%= item.name %>: <%= item.value %></li> <% } %> </ul> </div> </div> // And then display your category's posts looping on "posts" as you would do in the default "archive.html" template ...
Those are only simple examples.
Combining “template” and “template-args” filters is quite powerful and allows to implement nearly any template configuration and customization.
Thanks for reading and have fun with templates! 🙂
simple and useful plugin, but we need more support from your end.
Thank you for your feedback. We try through to have a good support through mails, doc and tutorials. You can also find demo themes fully commented on Github:
is posible adapt a new theme with Ionic Framework?
Hi Raul. It is not possible to use Ionic with WP-AppKit as Ionic uses AngularJS.
How can I use my wordpress theme for my app? I do not want to use a seperate WP-AppKit theme. The website should look approximately the same as the app.
Hi Jen, you can’t use your WordPress theme to build an app. A WordPress theme is done in PHP and hosted on a server and an hybrid app is done in JavaScript and hosted on a device. If you want to do that, you have to create an app which do only one thing: call your website into a custom browser.
Hi there,
I thing this is an amazing tool, i have a very silly question, can you tell me how to link local content in the app?.
I´m sorry about, its my first steps in programming.
Hi Guillermo, can you tell us what you mean by local content? Is this local to the app or to the website?
How to customize single post template from specific category?
You can use custom templates. Doc explains how to do it: https://uncategorized-creations.com/wp-appkit/doc/theme-api/#1714-custom-templates.
I have added this code and create gallery-template.html but the apps gone blank…
App.filter( ‘template’, function( template, current_screen ) {
if( TemplateTags.inCategory(‘gallery’, current_screen) ) {
template = ‘gallery-template’; //Don’t need .html here.
}
return template;
} );
You have a typo in your code: you should have isCategory instead of inCategory. (Please note that you can spot these common errors in the JavaScript console: hit F12 on your keyboard and have a look to the console.)
I am sorry sir, let me correect myself. I have post category called “gallery”. I want to make a specific template for single post which belong to “gallery” category.
How to achive that?
First you’ll have to add the post category list to data returned by the web service (by adding a .php file in the php folder of your theme). Then you’ll be able to use the template filter as you did for the archive template (in functions.js). I wrote a basic gist to show you how to do that: https://gist.github.com/blupu/27e4567aab17a800a6e306da128f9390.
I have copied the code below then I getting this error when i click on posts.
Uncaught TypeError: Cannot read property ‘indexOf’ of undefined
In your application panel, click on the Show Me Web Service Data button. It will show the JSON data returned by the web service. (You can prettify the output using the JSONView Chrome extension.) If you have added the PHP script to your theme, you should have a categories field for each post. Do you see it?
Lets say I have category term called ‘galeri-foto’.
I have add this code to my php script on my theme
function wpak_add_galeri_foto_template( $post_data, $post, $component ) {
$taxonomy = array( ‘category’ ); // We search for categories
$terms = get_the_terms( $post->ID, $taxonomy ); // Get the post categories
$cat_list = ”;
$cat_index = 0;
if ( $terms ) { // If post has at least one category
// Create a list of post category slugs separated by commas (= string)
foreach( $terms as $term_object ) {
if ( $cat_index != 0 ){
$cat_list .= ‘,’;
}
$cat_list .= $term_object->slug;
$cat_index++;
}
}
// Add category list to post data returned by the web service
$post_data[‘categories’] = $cat_list;
return $post_data; // Return the modified $post_data
}
add_filter( ‘wpak_post_data’, ‘wpak_add_galeri_foto_template’, 10, 3 );
Thne I added this code to function.js
App.filter( ‘template’, function( template, current_screen ) {
// Detect single
if ( current_screen.screen_type === ‘single’ ) {
// Get post categories (added in add-custom-data.php)
var post_categories = current_screen.data.post.categories;
// Call template for specific category’s slug
if ( post_categories.indexOf(‘galeri-foto’) !== -1 ){
template = ‘galeri-foto-template’;
}
}
return template;
});
But then I getting this on my service web data
Notice: Array to string conversion in /home/muhammad/public_html/balikbandung.com/wp-includes/taxonomy.php on line 3769
Notice: Array to string conversion in /home/muhammad/public_html/balikbandung.com/wp-includes/category-template.php on line 1178
Warning: Cannot modify header information – headers already sent by (output started at /home/muhammad/public_html/balikbandung.com/wp-includes/category-template.php:1178) in /home/muhammad/public_html/balikbandung.com/wp-content/plugins/wp-appkit-master/wp-appkit/lib/web-services/web-services.php on line 295
Warning: Cannot modify header information – headers already sent by (output started at /home/muhammad/public_html/balikbandung.com/wp-includes/category-template.php:1178) in /home/muhammad/public_html/balikbandung.com/wp-content/plugins/wp-appkit-master/wp-appkit/lib/web-services/web-services.php on line 296
Can you replace
$taxonomy = array( ‘category’ );
by$taxonomy = 'category';
and tell me if it solves your issue? (It seems that get_the_terms expects a string as second parameter.)Yeay It works….. Thanks, You really help me. I think you should include this example to your blog.
\o/ You’re right. That’s what we’re going to do. Thank you. (BTW I’ve slightly optimized the Gist.)
Thanks for your awesome plugin, how can I make sub menu for my app?
Thank you for using WP-AppKit 🙂 Unfortunatly I am afraid that submenus are not doable with the current UI. That’s something we’ll have to consider for the next versions. However you build a static menu in the app’s templates. Not an ideal solution but it might do the work.
I have a post type called “videos” i’m trying to create a template for its single page, So far i’ve create the template file called single-shows.php and also have pasted code below in my functions.js but its still working for me.
App.filter( ‘template’, function( template, current_screen) {
//Check the screen’s post type with “TemplateTags.isPostType()” :
//as we have to pass the current_screen as 3rd argument, we pass an empty post_id=0 as 2nd argument
//( because we don’t have to check a specific post ID here, just the post type ‘my-post-type’ ):
if( TemplateTags.isPostType(‘videos’, current_screen) ){
template = ‘single-videos’; //Don’t need .html here.
}
return template;
} );
is there something i should do, have to add it works with the default single.php file but not with mine single-shows.php
Hi,
You’re talking about single-shows.php file, here are my remarks:
– WP-AppKit themes are made with HTML files, so the extension should be “.html”, not “.php”
– you’re trying to include “single-videos.html”: is your file named “single-shows” or “single-videos”?
Plus, in your call to “TemplateTags.isPostType”, you didn’t pass any post ID before passing the “current_screen” object. You should have something like the following, just like in the comment above your code: “TemplateTags.isPostType(‘videos’, 0, current_screen)”
Finally, you can keep sending us email to our support address for this kind of request. We might not answer as quick here in the comments.
Impressive work, I’m happy to be able to create my mobile application, I just wanted to customize it as much as possible.
For now I do not know how to call the name of the category and its id
The_category and the_category_ID
Thank you!
Assuming you want to display the category name in the archive.html template, you can use
<%= list_title %>
. You’ll find détails in doc: https://uncategorized-creations.com/wp-appkit/doc/theme-api/#318-archive-html. Could tell us a bit more of what you want to do with the category ID?